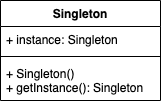
Singleton Pattern
2021, Aug 26
- Singleton là pattern tạo ra một instance duy nhất của một class
public class Singleton {
private static final Singleton instance = new Singleton();
public Singleton() {
//Do something in constructor
}
public static Singleton getInstance() {
return instance;
}
}
- Lazy singleton tạo instance khi được sử dụng
public class LazySingleton {
private static LazySingleton instance;
public LazySingleton() {
//Do something in constructor
}
public static LazySingleton getInstance() {
if (instance == null) {
instance = new LazySingleton();
}
return instance;
}
}
- Double checked singleton đảm bảo tạo singleton trong môi trường mutilthread
public class DoubleCheckedSingleton {
private static DoubleCheckedSingleton instance;
public DoubleCheckedSingleton() {
//Do something in constructor
}
public static DoubleCheckedSingleton getInstance() {
if (instance == null) {
synchronized (DoubleCheckedSingleton.class) {
if (instance != null) {
instance = new DoubleCheckedSingleton();
}
}
}
return instance;
}
}
- BillPugh singleton tạo singleton bằng cách sử dụng static inner class
public class BillPughSingleton {
private BillPughSingleton(){}
private static class SingletonHelper {
private static final BillPughSingleton INSTANCE = new BillPughSingleton();
}
public static BillPughSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
Source code ở đây